How to Show Automatic Internet Connection Offline Message in Flutter
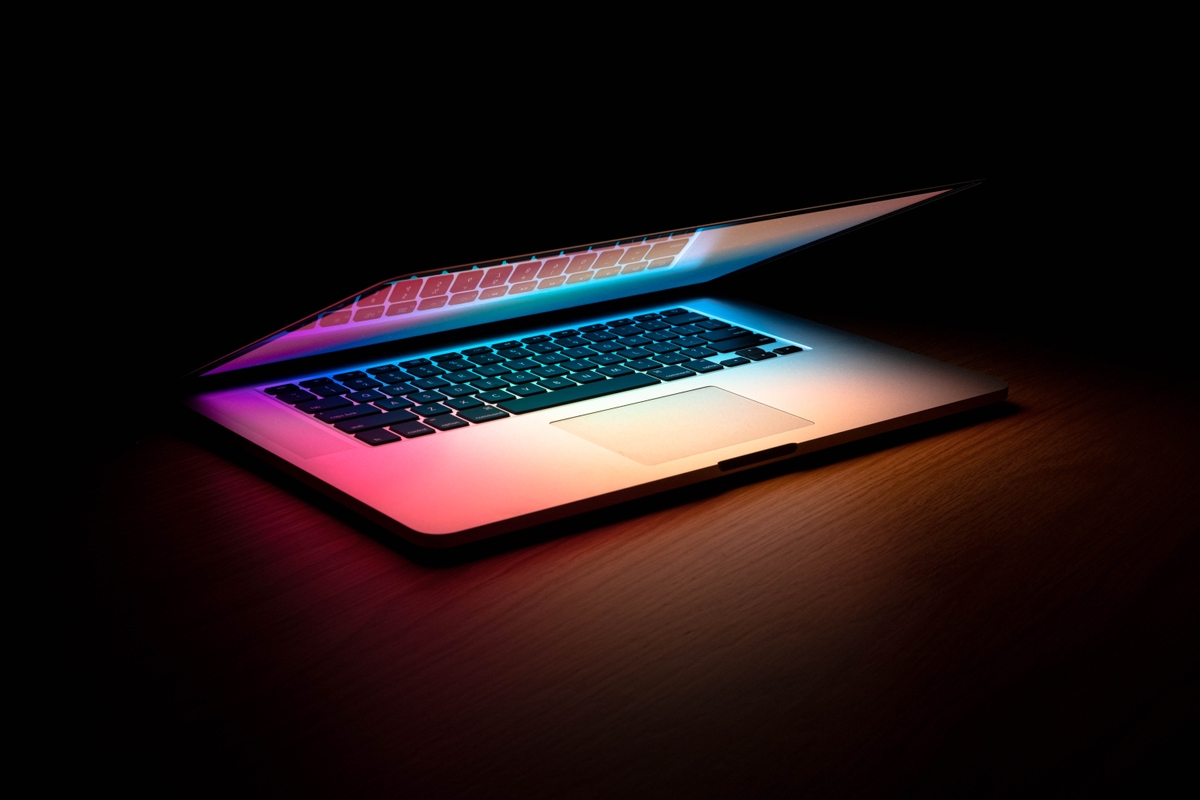
To implement an automatic internet connectivity message in your Flutter app, you’ll use the connectivity_plus package. This package allows you to determine the device’s connectivity status and react accordingly. Follow the steps below to add this functionality to your app.
Step 1: Add connectivity_plus to Your pubspec.yaml
First, include the connectivity_plus package in your pubspec.yaml file:
dependencies:
flutter:
sdk: flutter
connectivity_plus: ^6.0.3
Step 2: Implement the Connectivity Checker
Below is a complete example of how to create a widget that monitors connectivity status and displays an offline message when there is no internet connection.
import 'dart:async';
import 'package:connectivity_plus/connectivity_plus.dart';
import 'package:flutter/material.dart';
class CheckConnection extends StatefulWidget {
_CheckConnectionState createState() => _CheckConnectionState();
}
class _CheckConnectionState extends State<CheckConnection> {
StreamSubscription? internetConnection;
bool isOffline = false;
//set variable for Connectivity subscription listener
void initState() {
internetConnection =
Connectivity().onConnectivityChanged.listen((connectivityResult) {
if (connectivityResult.contains(ConnectivityResult.none)) {
//there is no any connection
setState(() {
isOffline = true;
});
} else if (connectivityResult.contains(ConnectivityResult.mobile) ||
connectivityResult.contains(ConnectivityResult.wifi)) {
//connection is from Wifi or Mobile
setState(() {
isOffline = false;
});
}
});
super.initState();
}
void dispose() {
super.dispose();
internetConnection?.cancel();
//cancel internet connection subscription after you are done
}
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text(
"Check Connection",
),
),
body: SingleChildScrollView(
child: Column(
children: [
Container(
child: errorMessage(
"No Internet Connection Available",
isOffline,
),
//to show internet connection message on isOffline = true.
),
Container(
margin: const EdgeInsets.all(
30,
),
width: double.infinity,
child: const Center(
child: Text(
"Check Connections",
style: TextStyle(
fontSize: 20,
),
),
),
),
],
),
),
);
}
Widget errorMessage(
String text,
bool show,
) {
//error message widget.
if (show == true) {
//if error is true then show error message box
return Container(
padding: const EdgeInsets.all(
10.00,
),
margin: const EdgeInsets.only(
bottom: 10.00,
),
color: Colors.red,
child: Row(children: [
Container(
margin: const EdgeInsets.only(
right: 6.00,
),
child: const Icon(
Icons.info,
color: Colors.white,
),
), // icon for error message
Text(
text,
style: const TextStyle(
color: Colors.white,
),
),
//show error message text
]),
);
} else {
//if error is false, return empty container.
return Container();
}
}
}
Conclusion
By following the above steps, you can seamlessly integrate an internet connectivity checker into your Flutter app. The connectivity_plus package helps you listen for connectivity changes and update the UI accordingly to inform the user when the device is offline. This enhances the user experience by providing immediate feedback on the network status.