Your Adventure in Appland: Crafting a "Hello World" App with Flutter in 2023
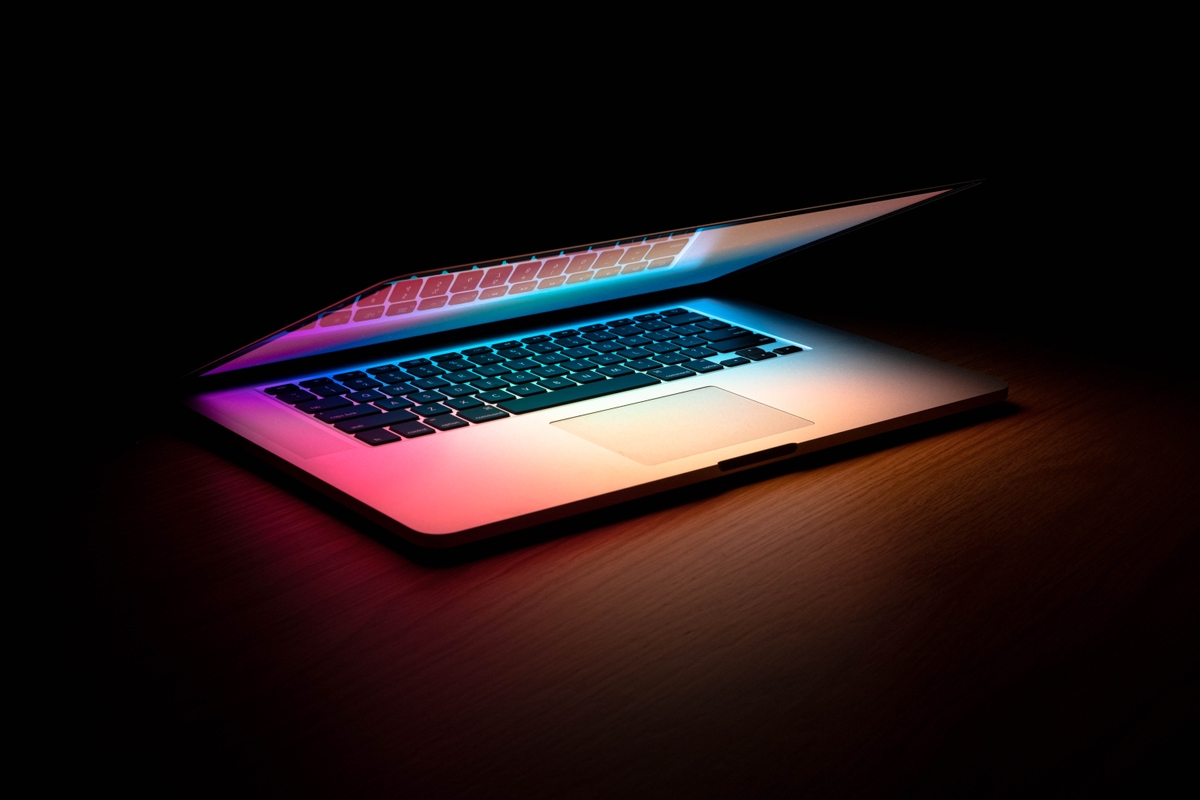
Greetings, aspiring app architects! Today, we embark on an exhilarating journey into the captivating world of mobile app development with Flutter. Whether you’re a code connoisseur eager to explore the latest trends or a complete coding novice with dreams of digital greatness, this step-by-step guide will walk you through creating your very own “Hello World” app using Flutter in the marvelous year of 2023.
- Step 1: Unleashing the Flutter Beast
- Step 2: Forging Your App’s Foundation
- Step 3: Mapping the App Terrain
- Step 4: Breathing Life into Your Creation
- Step 5: Mastering Platform Alchemy
- Step 6: Unleashing Your Creation in the Wild
- Step 7: Magic Touch - Adding Interactivity
- Step 8: Debugging Sorcery
- Step 9: Congratulations, Wizard of Flutterland!
Step 1: Unleashing the Flutter Beast
Before we dive in, let’s ensure you have Flutter roaring on your machine. Fret not, for taming this beast is easier than you think! Visit the official Flutter habitat at (https://flutter.dev) and follow the installation instructions tailored for your operating system. Don’t forget to saddle up with a code editor like Visual Studio Code or Android Studio to steer your development journey with ease.
Step 2: Forging Your App’s Foundation
With Flutter installed, let’s forge a new project from the depths of your creative mind. Fire up your chosen code editor and summon the “Create New Flutter Project” wizard. Bestow a name upon your masterpiece - for our quest, we’ll baptize it as “HelloWorld2023.”
Step 3: Mapping the App Terrain
As our creation takes form, let us chart its territory. Venture into the “lib” folder, where the heart of your app, “main.dart,” awaits. Here, we’ll sculpt the essence of our Hello World app’s user interface, breathing life into the void. Bid farewell to the existing code, and in its stead, forge the following foundation:
import 'package:flutter/material.dart';
void main() {
runApp(HelloWorldApp());
}
class HelloWorldApp extends StatelessWidget {
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(
title: Text('Hello World App - 2023'),
),
body: Center(
child: Text(
'Hello, World!',
style: TextStyle(fontSize: 24),
),
),
),
);
}
}
Step 4: Breathing Life into Your Creation
Let us breathe life into our creation! Seek out a simulator or emulator, loyal companions of the mobile app adventurer. Now, utter the sacred incantation in your terminal:
flutter run
Behold, as your Hello World app manifests on the simulator’s mystical screen, bridging the gap between imagination and reality.
Step 5: Mastering Platform Alchemy
Flutter grants us the sorcery to conjure apps for both Android and iOS, blurring the boundaries of the digital realm. Delve into the arcane arts of platform-specific customization. Embrace the native aesthetic, and your app shall blend harmoniously with the user’s enchanted device.
Step 6: Unleashing Your Creation in the Wild
Release your creation into the wilds of the physical world! To wield the power of real devices, arm yourself with developer options. For Android, unleash the hidden “USB debugging” within your phone’s settings. For iOS, become an esteemed member of the Apple Developer Program and traverse the enigmatic path they illuminate.
Step 7: Magic Touch - Adding Interactivity
Enchant your app with interactivity to captivate your users. Let’s enrich the “HelloWorldApp” class with the magic touch of user interaction:
class HelloWorldApp extends StatefulWidget {
_HelloWorldAppState createState() => _HelloWorldAppState();
}
class _HelloWorldAppState extends State<HelloWorldApp> {
String message = "Hello, World!";
void changeMessage() {
setState(() {
message = "Welcome to Flutter in 2023!";
});
}
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(
title: Text('Hello World App - 2023'),
),
body: Center(
child: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
Text(
message,
style: TextStyle(fontSize: 24),
),
RaisedButton(
onPressed: changeMessage,
child: Text('Change Message'),
),
],
),
),
),
);
}
}
Step 8: Debugging Sorcery
As the wisest of sages say, “With great magic comes great debugging.” Venture forth into the realm of testing with Flutter’s mystical tools. Unveil the secrets of “flutter test” for widget and integration testing. Utilize the arcane powers of debugging in your chosen editor to unveil and exorcise lurking gremlins.
Step 9: Congratulations, Wizard of Flutterland!
Congratulations, noble wizard! You’ve conjured your very own Hello World app with Flutter in the year 2023. But fear not, for this is but the prologue of your magical coding adventure. Unravel the mysteries of Flutter’s potent spells and libraries to craft even more captivating and spellbinding creations.
Remember, the journey of a thousand lines begins with a single “Hello, World!” incantation. Now, go forth and weave your coding magic, for the world eagerly awaits the wonders you shall conjure. Happy Fluttering, my fellow wizards!