Unlocking Flutter's Potential: Best Practices for Writing Clean Code
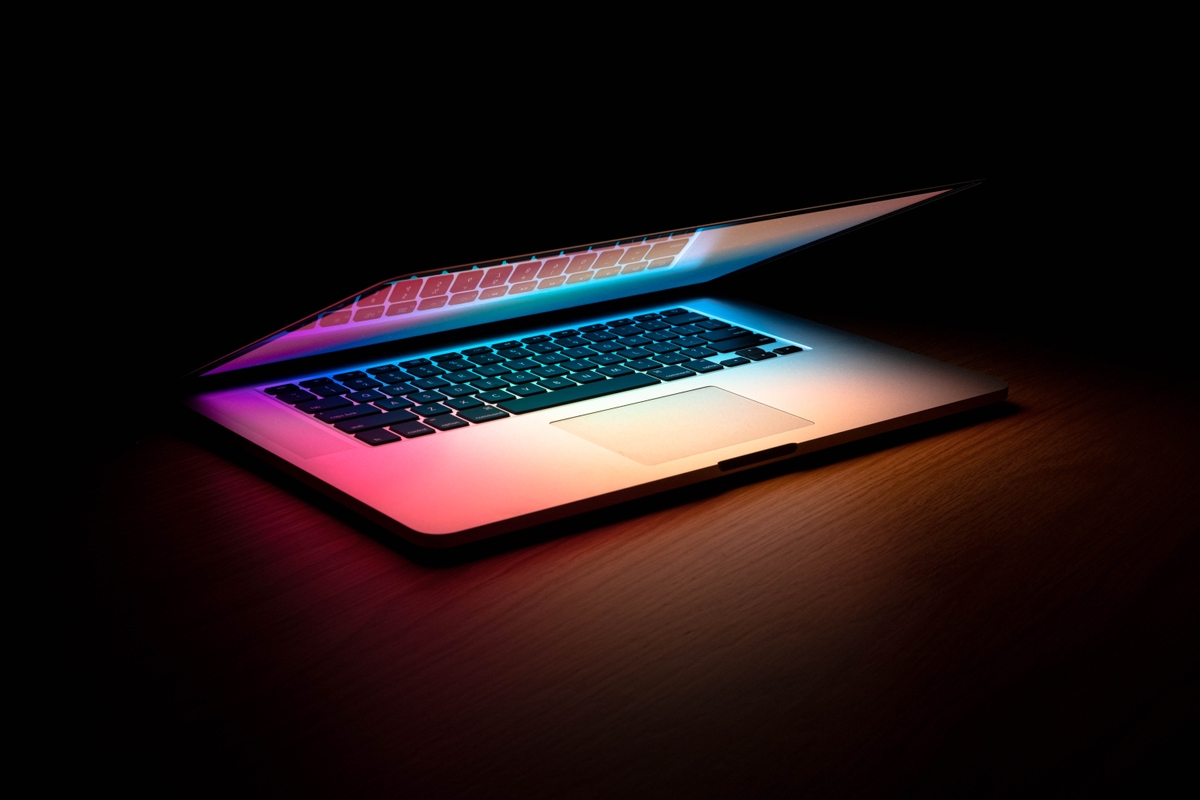
Flutter has gained immense popularity among developers due to its ability to create beautiful and high-performance mobile applications. However, as with any programming language or framework, writing clean and maintainable code is crucial for long-term success and productivity. Clean code not only enhances the readability and understandability of your codebase but also promotes collaboration, reduces bugs, and makes future modifications easier. In this article, we will explore some of the best practices for writing clean code in Flutter, along with examples in Dart.
Benefits of Clean Code
Before diving into the principles, let's briefly discuss the benefits of writing clean code:
-
Naming Conventions: Consistent and meaningful naming conventions improve code readability and understanding. Use descriptive names for variables, functions, and classes that accurately reflect their purpose and functionality.
Bad example:
var a = 10; // What does 'a' represent?
Pro example:
var itemCount = 10; // Descriptive variable name
-
Single Responsibility Principle (SRP): Each function and class should have a single responsibility or purpose. By adhering to SRP, your code becomes more modular and easier to maintain and test.
Bad example:
class User { // Contains user data, handles authentication, // and performs network requests }
Pro example:
class User { // Contains user data and handles authentication } class UserRepository { // Performs network requests related to users }
-
Don't Repeat Yourself (DRY): Avoid duplicating code by extracting common functionality into reusable functions or classes. DRY code reduces redundancy, improves maintainability, and enhances code readability.
Bad example:
void calculateSum() { // Perform addition // ... } void calculateProduct() { // Perform multiplication (similar logic as calculateSum) // ... }
Pro example:
int calculateSum(int a, int b) { return a + b; } int calculateProduct(int a, int b) { return a * b; }
-
Keep Functions and Classes Small: Breaking down functions and classes into smaller units promotes code readability, reusability, and easier testing. Each function and class should have a clear and concise purpose.
Bad example:
void processUserData() { // 100 lines of code }
Pro example:
void fetchData() { // Fetch data from the server } void processData() { // Process fetched data } void displayData() { // Display processed data }
-
Use Descriptive Comments: Comments should be used sparingly and only when necessary to clarify complex logic or provide additional context. Avoid redundant or misleading comments.
Bad example:
// Increment counter by 1 counter++;
Pro example:
counter++; // Increase the counter by 1
-
Follow Code Formatting Guidelines: Consistent code formatting improves readability and maintainability. Adhere to the style guide recommended by the Dart language and Flutter community, such as using proper indentation, line breaks, and spacing.
Bad example:
void calculateDiscount(int price,double discount) { if(discount>0.5){ price = price - price * discount; } else{price=price;} }
Pro example:
void calculateDiscount(int price, double discount) { if (discount > 0.5) { price = price - price * discount; } else { price = price; } }
-
Code Readability: Write code that is easy to read and understand. Use meaningful variable and function names, break down complex logic into smaller parts, and prioritize clarity over cleverness.
Bad example:
var x = a + b * (c - d) / e;
Pro example:
var totalPrice = basePrice + taxRate * (discountedPrice - previousPrice) / exchangeRate;
-
Error Handling: Proper error handling enhances the reliability and robustness of your code. Use try-catch blocks to handle exceptions, provide informative error messages, and handle potential failure scenarios gracefully.
Bad example:
void fetchData() { try { // Fetch data } catch (e) { print('An error occurred'); } }
Pro example:
void fetchData() { try { // Fetch data } catch (e) { print('Failed to fetch data: $e'); } }
-
Unit Testing: Write unit tests to verify the correctness of your code and catch potential issues early. Test individual functions, classes, and modules to ensure they behave as expected.
Bad example:
// No unit tests written
Pro example:
void testCalculateSum() { expect(calculateSum(2, 3), equals(5)); }
-
Consistent Code Structure: Maintain a consistent code structure throughout your project. Use the same folder structure, naming conventions, and code organization principles to make navigation and understanding easier for you and other developers.
Bad example:
lib/ screens/ home_screen.dart profile_screen.dart utils/ common.dart helper.dart
Pro example:
lib/ screens/ home/ home_screen.dart profile/ profile_screen.dart utils/ common_utils.dart helper_utils.dart
-
Code Documentation: Document your code using comments and docstrings to explain the purpose, inputs, outputs, and any potential side effects of functions, classes, and methods. Well-documented code is easier to understand and maintain.
Bad example:
// Function to calculate the sum void calculateSum() { // ... }
Pro example:
/// Calculates the sum of two numbers. /// /// Returns the sum of [a] and [b]. int calculateSum(int a, int b) { return a + b; }
-
Code Review: Embrace code reviews as an essential part of your development process. Collaborate with other developers to review and provide feedback on your code, ensuring adherence to best practices and identifying potential issues.
Bad example:
// No code review conducted
Pro example:
// Code review feedback incorporated
By following these principles, you can unlock Flutter's full potential and create clean, maintainable, and high-quality code. Remember, writing clean code is an ongoing effort that requires continuous learning and improvement. Strive to apply these best practices consistently, and you will reap the rewards in the long run.
Now that we understand the benefits of clean code, let's delve into some critical principles and best practices, accompanied by bad, good, and perfect examples in Dart.
- Avoid Deeply Nested Code: One of the fundamental principles of clean code is to avoid deeply nested code structures. Deeply nested code can quickly become challenging to read, understand, and maintain. Let's look at an example:
Bad Example:
Widget build(BuildContext context) {
return Container(
child: Column(
children: [
Expanded(
child: ListView.builder(
itemCount: 10,
itemBuilder: (BuildContext context, int index) {
return Text('Item $index');
},
),
),
],
),
);
}
Pro Example:
Widget build(BuildContext context) {
return Container(
child: Expanded(
child: ListView.builder(
itemCount: 10,
itemBuilder: (BuildContext context, int index) {
return Text('Item $index');
},
),
),
);
}
By reducing unnecessary nesting, the code becomes more readable and less prone to errors.
- Avoid Magic Numbers and Strings: Magic numbers and strings are hardcoded values that lack proper explanation or context. It is considered a best practice to avoid using them in your code. Here's an example:
Bad Example:
if (statusCode == 200) {
print('Success');
}
Pro Example:
const int successStatusCode = 200;
if (statusCode == successStatusCode) {
print('Success');
}
By using a named constant instead of a magic number, the code becomes more self-explanatory and easier to maintain.
- Meaningful Variable Scope: To improve code readability and maintainability, it is important to define variable scopes appropriately. Variables should have the narrowest scope possible while still fulfilling their purpose. Let's see an example:
Bad Example:
void processOrder() {
String orderID;
// Code to process order
print(orderID);
}
Pro Example:
void processOrder() {
String orderID;
// Code to process order
print(orderID);
}
By defining the orderID variable within the scope where it is actually used, we improve code clarity and reduce the chances of accidentally misusing or modifying the variable.
- Avoid Large Classes: Large classes can be overwhelming and difficult to comprehend. It is essential to keep classes focused on a single responsibility and avoid excessive complexity. Here's an example:
Bad Example:
class UserManager {
// Lots of properties and methods...
}
Pro Example:
class UserManager {
// Focused properties and methods...
}
By breaking down large classes into smaller, more focused units, we improve code organization and make it easier to maintain and test.
- Separation of Concerns (SoC): The principle of Separation of Concerns advocates for dividing code into distinct sections, each handling a specific responsibility. By separating different concerns, we enhance code modularity and reusability. Let's look at an example:
Bad Example:
class LoginPage extends StatefulWidget {
// Widget code...
void validateForm() {
// Form validation code...
}
void submitForm() {
// Form submission code...
}
}
Pro Example:
class LoginPage extends StatefulWidget {
// Widget code...
void validateForm() {
// Form validation code...
}
}
class LoginForm {
void submitForm() {
// Form submission code...
}
}
By separating the form validation and submission logic into separate classes, we improve code organization and make it easier to maintain and test.
- Avoid Long Parameter Lists: Long parameter lists can make code harder to read and maintain. It is advisable to limit the number of parameters a method or function requires. Here's an example:
Bad Example:
void updateUser(String firstName, String lastName, String email, String address, int age, String phoneNumber) {
// Code to update user...
}
Pro Example:
class User {
String firstName;
String lastName;
String email;
String address;
int age;
String phoneNumber;
}
void updateUser(User user) {
// Code to update user...
}
By passing a single object or struct instead of multiple parameters, the code becomes more concise and avoids potential errors due to parameter order or missing values.
- Meaningful and Consistent Formatting: Consistent formatting and naming conventions contribute to code readability and maintainability. Adopting a standard formatting style and adhering to it throughout the codebase is crucial. Here's an example:
Bad Example:
void processOrder() {
String orderID;
// Code to process order
print(orderID);
}
Pro Example:
void processOrder() {
String orderId;
// Code to process order
print(orderId);
}
By using proper indentation, consistent spacing, and adhering to a consistent naming convention (e.g., camel case), we improve code readability and make it easier for others to understand and contribute to the codebase.
- Avoid Global Variables: Global variables make it challenging to reason about code behavior and can introduce unexpected side effects. It is recommended to limit the use of global variables and instead encapsulate state within the appropriate classes or scopes. Let's see an example:
Bad Example:
String authToken;
void fetchUserData() {
// Code to fetch user data using authToken...
}
Pro Example:
class AuthTokenManager {
String authToken;
void fetchUserData() {
// Code to fetch user data using authToken...
}
}
By encapsulating the authToken within a class, we limit its visibility and reduce the risk of unintended modifications or conflicts.
- SOLID Principles: The SOLID principles (Single Responsibility, Open-Closed, Liskov Substitution, Interface Segregation, and Dependency Inversion) are essential guidelines for writing maintainable and extensible code. Adhering to these principles promotes modular design and enhances code quality. Let's consider the Single Responsibility Principle (SRP):
Bad Example:
class User {
void register() {
// Code for user registration...
}
void sendNotification() {
// Code to send notification...
}
}
Pro Example:
class UserRegistration {
void register() {
// Code for user registration...
}
}
class NotificationSender {
void sendNotification() {
// Code to send notification...
}
}
By separating the responsibilities of user registration and notification sending into distinct classes, we adhere to the SRP and improve code organization and maintainability. Learn More
-
Continuous Refactoring: Refactoring is an ongoing process of improving code quality without changing its external behavior. Regularly reviewing and refactoring code allows for better design, reduced complexity, and improved performance. It is crucial to allocate time for refactoring during the development process.
-
Use Tools and Static Analysis: Leveraging tools and static analysis can help identify potential issues, enforce code style guidelines, and catch errors before they manifest. Tools like linters and static analyzers (e.g., Dart Analyzer, Flutter Inspector) can be integrated into development workflows to ensure code quality.
-
Consider Performance: Efficient code plays a significant role in delivering a smooth user experience. It is important to consider performance implications when writing Flutter code. Avoid unnecessary computations, optimize resource usage, and utilize caching and memoization techniques when appropriate.
-
Meaningful Method Signatures: Well-designed method signatures communicate their intent and expectations clearly. Use descriptive and concise names for methods, and ensure that the parameters and return types accurately reflect the purpose of the method. Let's consider an example:
Bad Example:
List<int> f(List<int> a, List<int> b) {
// Code...
}
Pro Example:
List<int> mergeLists(List<int> listA, List<int> listB) {
// Code...
}
By using meaningful names and providing clear context in method signatures, we improve code readability and maintainability.
-
Avoid Side Effects: Side effects occur when a function or method modifies state outside of its own scope. Minimizing side effects makes code more predictable, testable, and easier to reason about. It is advisable to design functions and methods to be pure and free of unexpected side effects.
-
Use Design Patterns Appropriately: Design patterns provide proven solutions to common software design problems. Familiarize yourself with commonly used design patterns and apply them appropriately in your Flutter code. Examples of design patterns include the Singleton, Observer, and Factory patterns.
-
Keep Code Modular and Reusable: Modular code allows for better organization, maintainability, and code reuse. Aim to break down functionality into smaller, self-contained modules that can be easily understood and reused in different parts of your application.
-
Avoid Complex Conditional Logic: Complex conditional logic can make code difficult to read, understand, and maintain. Strive to simplify conditional statements by using techniques such as early returns, guard clauses, and ternary operators.:
Bad Example:
void processOrder(Order order) {
if (order != null) {
if (order.status == OrderStatus.PENDING) {
if (order.paymentStatus == PaymentStatus.PAID) {
if (order.items.isNotEmpty()) {
// Process the order...
} else {
// Handle empty order items...
}
} else {
// Handle unpaid order...
}
} else {
// Handle non-pending order...
}
} else {
// Handle null order...
}
}
Pro Example:
void processOrder(Order order) {
if (order == null) {
// Handle null order...
return;
}
if (order.status != OrderStatus.PENDING) {
// Handle non-pending order...
return;
}
if (order.paymentStatus != PaymentStatus.PAID) {
// Handle unpaid order...
return;
}
if (order.items.isEmpty()) {
// Handle empty order items...
return;
}
// Process the order...
}
-
Version Control and Git Best Practices: Utilize version control systems like Git and follow best practices such as using meaningful commit messages, creating branches for new features or bug fixes, and regularly pushing changes to a remote repository. Proper version control enables collaboration, facilitates code review, and provides a safety net for code changes.
-
Minimize Method and Function Length: Long methods and functions tend to be more challenging to understand and maintain. Aim to keep methods and functions concise, focusing on a single task. Consider breaking down complex functionality into smaller, more manageable units
Bad Example:
void processOrder(Order order) {
// Long and complex code...
}
Pro Example:
void processOrder(Order order) {
validateOrder(order);
calculateTotal(order);
generateInvoice(order);
// ...
}
void validateOrder(Order order) {
// Validation logic...
}
void calculateTotal(Order order) {
// Calculation logic...
}
void generateInvoice(Order order) {
// Invoice generation logic...
}
-
Avoid Commented-Out Code: Commented-out code fragments clutter the codebase and can mislead developers who encounter them. Remove unnecessary commented-out code and rely on version control history to retrieve earlier versions if needed.
-
Use Dart Language Features Effectively: Familiarize yourself with the features and idioms of the Dart programming language and utilize them effectively to write concise, expressive, and readable code. Examples include named parameters, cascades, null safety, and async/await.
-
Code Ownership and Collaboration: Collaborative development requires clear ownership of code sections. Clearly define responsibilities and roles within your team, and establish guidelines for code reviews, pair programming, and knowledge sharing. Encourage open communication and constructive feedback to improve code quality and foster a collaborative environment.
-
Keep Dependencies Updated: Regularly update your project dependencies to leverage the latest bug fixes, performance improvements, and features. Monitor for updates and follow best practices for dependency management, ensuring that your application remains secure and up-to-date.
Bonus Principles:
- Avoid Fragile Base Class (FBC) Problem: The Fragile Base Class problem occurs when changes in the base class unintentionally affect derived classes, leading to unexpected bugs and maintenance headaches. To avoid this, prefer composition over inheritance whenever possible. Let's consider an example:
Bad Example:
class Animal {
void makeSound() {
print('Animal sound');
}
}
class Dog extends Animal {
void makeSound() {
print('Bark');
}
}
Pro Example:
class Animal {
void makeSound() {
print('Animal sound');
}
}
class Dog {
Animal _animal;
Dog(this._animal);
void makeSound() {
_animal.makeSound();
}
}
- Avoid Feature Envy: Feature Envy occurs when a method or function excessively relies on data or methods of another class, indicating a poor distribution of responsibilities. It is best to keep code modular and adhere to the single responsibility principle. Consider the following example:
Bad Example:
class User {
String name;
String email;
void sendEmail(String message) {
// Sending email using the email field
}
void printName() {
print(name);
}
}
Pro Example:
class User {
String name;
String email;
void sendEmail(String message) {
// Sending email using the email field
}
}
class UserPrinter {
void printName(User user) {
print(user.name);
}
}
- Avoid Large Widgets and Functions:
Large widgets and functions are challenging to understand, maintain, and test. Breaking them down into smaller, reusable components improves code readability and promotes code reuse. Let's examine an example:
Bad Example:
class HomePage extends StatelessWidget {
Widget build(BuildContext context) {
return Scaffold(
// A large number of nested widgets and complex logic
);
}
}
Pro Example:
class HomePage extends StatelessWidget {
Widget build(BuildContext context) {
return Scaffold(
body: Column(
children: [
HeaderWidget(),
ContentWidget(),
FooterWidget(),
],
),
);
}
}
- Consider Flutter Widget Lifecycle: Understanding the Flutter widget lifecycle is crucial for managing resources and state. It ensures that resources are efficiently utilized and prevents memory leaks. Let's see an example of handling the widget lifecycle correctly:
Bad Example:
class MyWidget extends StatefulWidget {
_MyWidgetState createState() => _MyWidgetState();
}
class _MyWidgetState extends State<MyWidget> {
StreamSubscription _subscription;
void initState() {
super.initState();
_subscription = fetchData().listen((data) {
// Handle data
});
}
void dispose() {
_subscription.cancel();
super.dispose();
}
Widget build(BuildContext context) {
return Container();
}
}
Pro Example:
class MyWidget extends StatefulWidget {
_MyWidgetState createState() => _MyWidgetState();
}
class _MyWidgetState extends State<MyWidget> {
late StreamSubscription _subscription;
void initState() {
super.initState();
_subscription = fetchData().listen((data) {
// Handle data
});
}
void dispose() {
_subscription.cancel();
super.dispose();
}
Widget build(BuildContext context) {
return Container();
}
}
- Avoid Duplicate Code: Duplicating code leads to maintenance nightmares and increases the likelihood of introducing bugs. It is crucial to identify common code patterns and extract them into reusable methods or classes. Consider the following example:
Bad Example:
void logInUser(String email, String password) {
// Validation and authentication logic
// ...
print('User logged in successfully');
}
void signUpUser(String email, String password) {
// Validation and authentication logic
// ...
print('User signed up successfully');
}
Pro Example:
void authenticateUser(String email, String password) {
// Validation and authentication logic
}
void logInUser(String email, String password) {
authenticateUser(email, password);
print('User logged in successfully');
}
void signUpUser(String email, String password) {
authenticateUser(email, password);
print('User signed up successfully');
}
- Avoid Hardcoding Values: Hardcoding values makes code inflexible and difficult to maintain. Instead, use constants or configuration files to store values that are likely to change. Let's look at an example:
Bad Example:
void showSnackBar(BuildContext context) {
ScaffoldMessenger.of(context).showSnackBar(
SnackBar(
content: Text('Error: Something went wrong'),
),
);
}
Pro Example:
const String errorMessage = 'Error: Something went wrong';
void showSnackBar(BuildContext context) {
ScaffoldMessenger.of(context).showSnackBar(
SnackBar(
content: Text(errorMessage),
),
);
}
- Consider Error Handling: Proper error handling improves the stability of the application and provides meaningful feedback to users. Always handle potential exceptions and errors gracefully. Here's an example:
Bad Example:
void fetchData() {
try {
// Fetch data from the network
} catch (e) {
print('Error: $e');
}
}
Pro Example:
void fetchData() {
try {
// Fetch data from the network
} catch (e) {
handleError(e);
}
}
void handleError(dynamic error) {
// Log, display an error message,
//or perform appropriate actions
}
Conclusion:
Adopting best practices for writing clean code is essential for unlocking the full potential of Flutter. By avoiding the Fragile Base Class problem, Feature Envy, large widgets and functions, and other common pitfalls, you can create maintainable, readable, and robust code. Remember to consider the Flutter widget lifecycle, eliminate duplicate code, avoid hardcoding values, and handle errors gracefully. By following these guidelines, you can enhance your Flutter development workflow and create high-quality applications. Happy coding!