Flutter Debug Banner Removal: Best Practices and Techniques
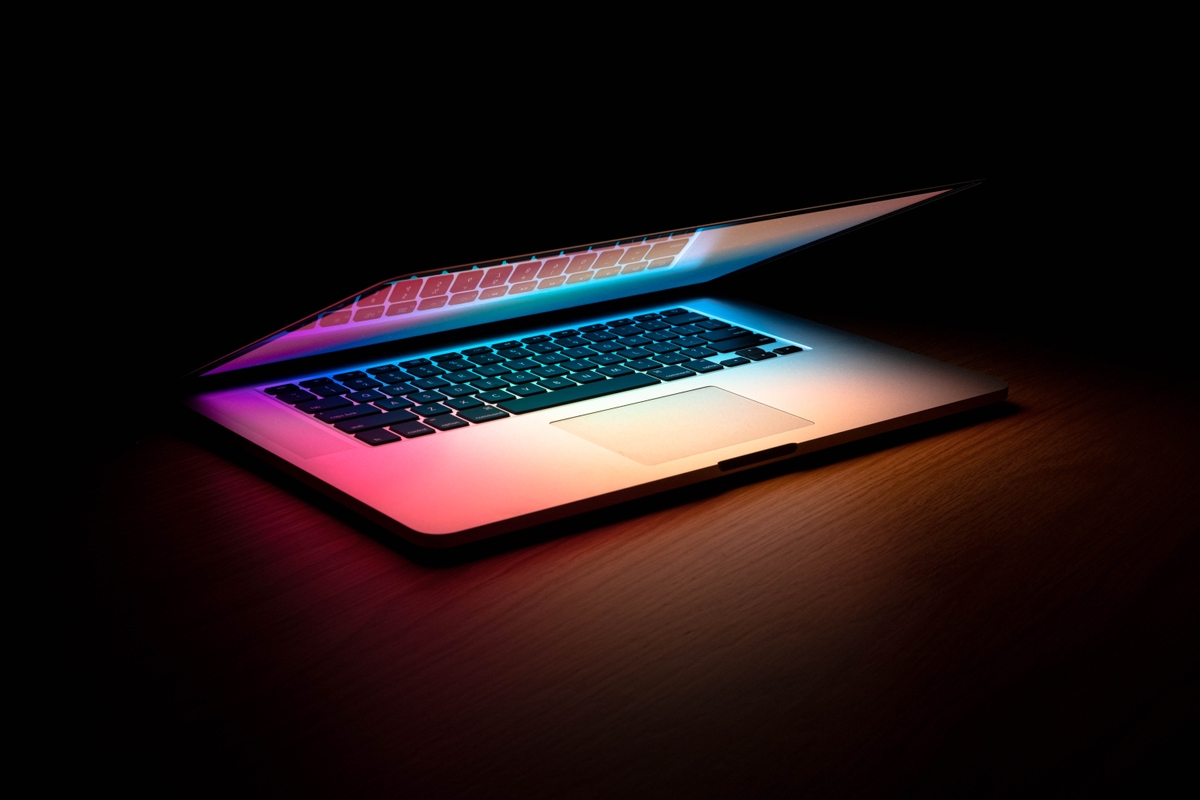
Introduction
When developing Flutter applications, it is common to see a red “Debug” banner at the top right corner of the screen. While this banner is useful during the development phase, it is not desirable in the final release or production version of your app. Removing the debug banner is a crucial step in providing a polished and professional user experience. In this tutorial, we will explore the best practices and techniques for removing the debug banner in Flutter applications.
Prerequisites
Before we proceed, ensure that you have the following prerequisites in place:
- Flutter SDK installed on your machine.
- A Flutter project set up and ready for development.
Step 1: Remove Debug Banner in Release Mode
To remove the debug banner, we need to disable it in the release version of our Flutter application. Follow these steps:
1.Open the lib/main.dart file in your Flutter project.
2.Locate the MaterialApp widget that wraps your application.
3.Add the debugShowCheckedModeBanner property to the MaterialApp widget and set it to false.
Here’s an example:
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
Widget build(BuildContext context) {
return MaterialApp(
debugShowCheckedModeBanner: false, // Add this line
home: MyHomePage(),
);
}
}
With debugShowCheckedModeBanner set to false, the debug banner will not be displayed in the release version of your app.
Bonus: Removing Debug Banner for Specific Builds
In some cases, you may want to remove the debug banner only for specific build flavors, such as release builds. Flutter allows you to define custom build flavors in your pubspec.yaml file. To remove the debug banner for a specific flavor, follow these steps:
1.Open your pubspec.yaml file.
2.Locate the flutter section and add a flavor configuration with a name of your choice.
For example:
flutter:
flavorDimensions: []
flavors:
flavor_name: // Add additional configuration here
- Specify the desired build flavor configuration. For example, to remove the debug banner for the release flavor, modify the flavor_name configuration as follows:
flutter:
flavorDimensions: []
flavors:
release: // Additional configuration
- Open the lib/main.dart file and modify the MaterialApp widget to conditionally disable the debug banner based on the current flavor.
Here’s an example:
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
Widget build(BuildContext context) {
bool removeDebugBanner = false;
// Check the current flavor and set removeDebugBanner accordingly
if (const bool.fromEnvironment('dart.vm.product')) {
// Running in release flavor
removeDebugBanner = true;
}
return MaterialApp(
debugShowCheckedModeBanner: !removeDebugBanner, // Toggle the debug banner
home: MyHomePage(),
);
}
}
With this setup, the debug banner will be removed only for the specified build flavor, in this case, the release flavor.
Conclusion
In this tutorial, we have learned how to remove the debug banner in Flutter applications. We explored different techniques, including disabling the debug banner in the release version and configuring build flavors. By following these best practices, you can ensure that your Flutter app provides a seamless and professional user experience in the final release version.
Remember to thoroughly test your application after removing the debug banner to confirm that everything functions as expected. Happy coding!